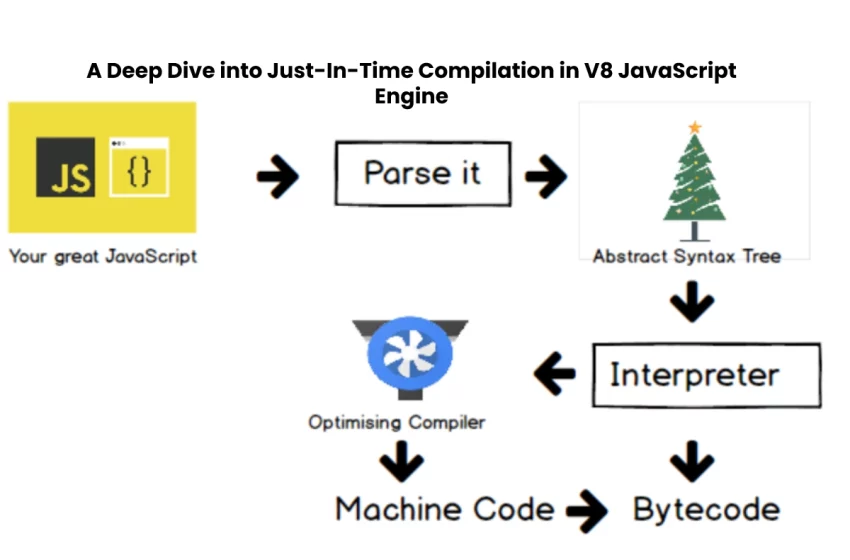
A Deep Dive into Just-In-Time Compilation in V8 JavaScript Engine
Are you a developer looking to optimize your code? Or perhaps an end-user hoping for a faster browsing experience? Either way, you’ve likely come across the term “just-in-time compilation” (JIT) in your search for heightened efficiency. But what exactly is JIT, and how does it work within V8, one of the most popular JavaScript engines? Can you use the swift package repository, like in this JFrog use-case guide, to fine-tune your code?
Table of Contents
Understanding Just-In-Time Compilation
First, let’s start with the basics. Just-In-Time Compilation is a technique used by programming language implementations to improve their performance by dynamically compiling code on the fly rather than ahead of time (AOT). Essentially, JIT compilers will analyze a program’s patterns of execution in real-time and generate optimized machine code on the spot rather than converting the entire program to machine code before execution.
V8, the JavaScript engine developed by Google, uses JIT compilation to translate JavaScript code into machine code that can run on the computer’s processor. But how does it achieve this, and what steps are involved?
The Three-Step JIT Compilation Process
Three main steps are involved in JIT compilation and optimization in V8: parsing and compilation, function optimization, and code generation.
- Parsing and Compilation: The first step of JIT involves parsing the JavaScript code and translating it into an intermediate representation (IR). The IR is then compiled into machine code, known as “unoptimized code.” This unoptimized code is still faster than interpreted code but is not yet fully optimized.
- Function Optimization: The second step involves analyzing and optimizing individual functions within the code. V8’s compiler uses a technique called “Inline Caching,” which caches the types of objects passed to a function to be used in subsequent calls. The more a function is called, the more optimized it becomes.
- Code Generation: The final step of JIT generates fully optimized machine code. V8 uses several techniques for optimizing code, such as “hidden classes” and “inline caching.” Hidden classes allow V8 to allocate memory more efficiently and reduce the frequency of garbage collection. Inline caching optimizes the lookup of object properties, reducing the time and resources needed for property accesses.
Benefits and Drawbacks of JIT Compilation
Just-In-Time Compilation is a powerful tool for enhancing performance, and V8 takes full advantage of the technology. But it’s not without drawbacks. JIT compilation comes with inevitable tradeoffs, such as increased memory usage and slower startup times due to the extra overhead involved in compiling and optimizing code.
On the other hand, the benefits of JIT compilation far outweigh the drawbacks. V8 can significantly improve performance and reduce latency by dynamically translating code into optimized machine instructions. This makes web applications run smoother and faster, resulting in a better user experience.
In addition to improved performance, JIT-compiled code is also more secure than traditional interpreted code because it’s harder for hackers to reverse-engineer optimized machine instructions.
However, in recent years, JIT has been criticized for potentially exposing users to security vulnerabilities. Because JIT compilers execute code dynamically, they can create paths for attackers to exploit, such as buffer overflow attacks or code injection attacks. To mitigate these risks, researchers have explored various strategies for improving the security of JIT compilation, such as reducing exposure to memory leaks or isolating JIT code in a separate process.
Strategies for Improving JIT Efficiency
Despite the potential risks, JIT remains a critical component of modern JavaScript engines. To minimize the drawbacks of JIT, developers have explored various strategies for improving the efficiency of JIT compilation.
Here are some strategies for improving the performance of JIT-compiled code:
- Monitor Performance: To ensure your code is optimized, use profiling tools to monitor its performance and identify any bottlenecks or inefficiencies. This will help you locate areas where performance can be improved and optimize them accordingly.
- Use an Ahead-of-Time Compiler: If you’re concerned about security risks, consider using an ahead-of-time (AOT) compiler instead of a JIT compiler. AOT compilers can provide comparable performance with fewer risks.
- Optimize Memory Usage: Memory is one of the critical resources in optimizing code for execution, and inefficient memory usage can drastically reduce performance. Make sure to optimize memory usage as much as possible to ensure your code runs efficiently.
- Identify Hot Functions: JIT compilers will typically prioritize the optimization of functions that are frequently called, known as “hot functions.” Identifying and optimizing these hot functions can help speed up execution time.
- Cache Optimized Code: Once a function has been optimized, it’s a good idea to cache the code so it doesn’t need to be recompiled. This will help ensure that your code is running smoothly and efficiently.